1. Introduction to React
- React Official Docs
The best place to start if you’re new to React. It covers the basics of components, JSX, and state.
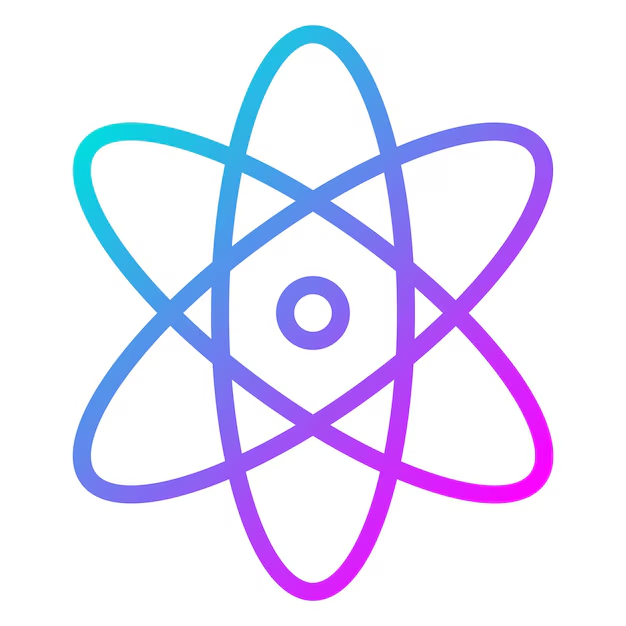

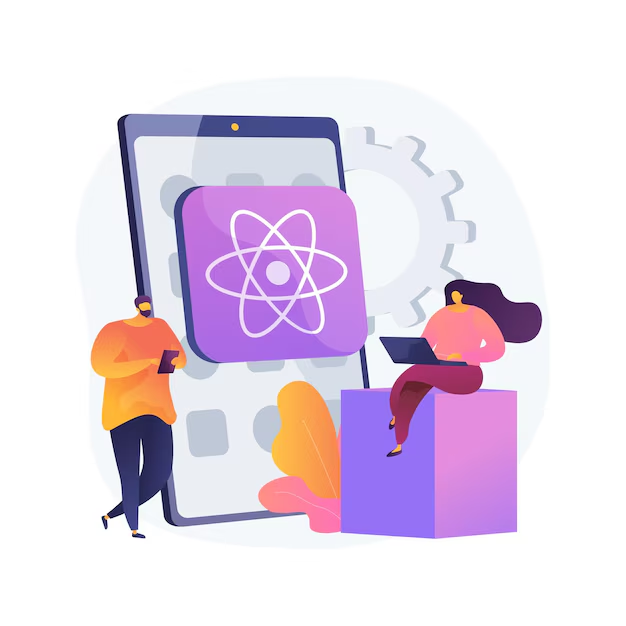
2. Understanding JSX
- Article: What is JSX in React?
Learn how JSX simplifies UI creation and how it translates to regular JavaScript.
3. React Hooks
- Article: A Complete Guide to React Hooks
Explains hooks likeuseState
,useEffect
, and custom hooks with practical examples. - Deep Dive into useEffect: How to Master React’s useEffect
4. State Management
- Article: React State Management: A Practical Guide
Discusses built-in React state, Context API, and popular libraries like Redux and Zustand.
5. React with TypeScript
- Article: React and TypeScript: A Beginner’s Guide
A great resource to integrate static typing into React for better code reliability.
6. Performance Optimization
- Article: Optimizing React Applications
Covers techniques like memoization, lazy loading, and code splitting.
7. Component Design Best Practices
- Article: How to Write Better React Components
Tips on making your components reusable, readable, and maintainable.
8. React Router
- Article: Mastering React Router
Learn how to handle navigation and routing in React apps using React Router.
9. Server-Side Rendering (SSR) with Next.js
- Article: Why Use Next.js with React
An introduction to Next.js, which extends React for building SSR and static sites.
10. Testing in React
- Article: Testing React Applications with Jest and React Testing Library
A practical guide to testing React components effectively.